6.4 চি-ত এৰে উপাদানসমূহ প্ৰাপ্ত কৰা
Till now we have seen how to declare an array in the C programming language. We will now see how we can access each element of an array – how to take input, how to display etc.
এতিয়ালৈকে আমি চি প্ৰগ্ৰামিং ভাষাত এটা এৰে কেনেদৰে ঘোষণা কৰিব লাগে দেখিছোঁ। আমি এতিয়া চাম কেনেদৰে আমি এৰেএটাৰ প্ৰতিটো উপাদান প্ৰাপ্ত কৰিব পাৰোঁ – ইনপুট কেনেদৰে ল’ব লাগে, কেনেদৰে প্ৰদৰ্শন কৰিব লাগে ইত্যাদি।
We have already seen that each element in an array has a specific location or index. We can use this in order to access a particular element. Figure 6.2 shows this clearly.
আমি ইতিমধ্যে দেখিছোঁ যে এটা এৰেৰ প্ৰতিটো উপাদানৰ এটা নিৰ্দিষ্ট অৱস্থান বা সূচক আছে। আমি এটা নিৰ্দিষ্ট উপাদানত প্ৰৱেশ কৰিবলৈ ইয়াক ব্যৱহাৰ কৰিব পাৰোঁ। চিত্ৰ 6.2-য়ে এইটো স্পষ্টভাৱে দেখুৱায়।
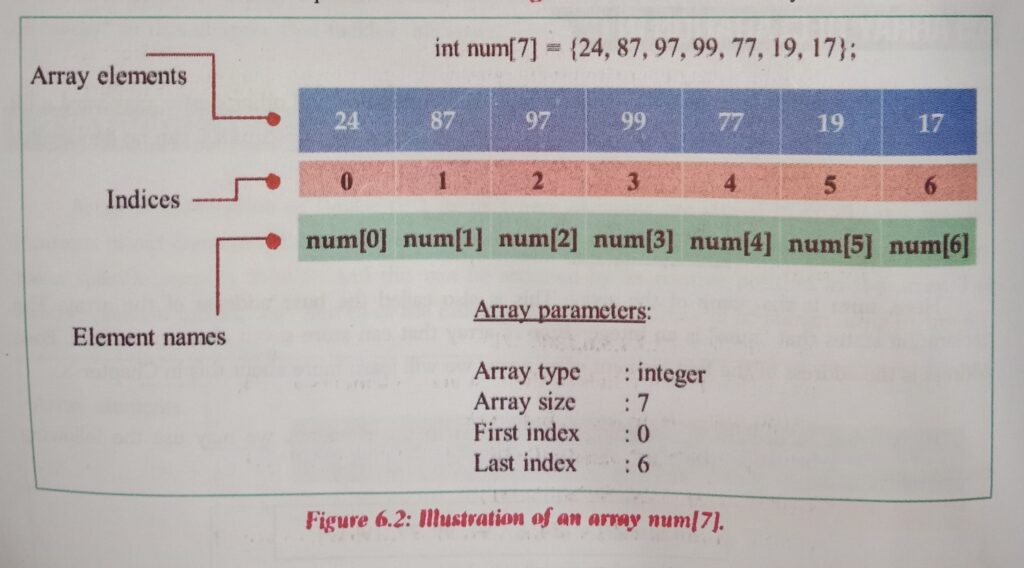
First element in the array can be referred as num[0]. The second one can be referred as num[1] and so on. In general, any individual element can be referred as num[id], where num is the name of the array and id is the index of that element in the array.
এৰেৰ প্ৰথম উপাদানটোক সংখ্যা[0] বুলি ক’ব পাৰি। দ্বিতীয়টোক সংখ্যা[1] ইত্যাদি বুলি ক’ব পাৰি। সাধাৰণতে, যিকোনো পৃথক উপাদানক সংখ্যা[আইডি] বুলি ক’ব পাৰি, য’ত সংখ্যা হৈছে এৰেৰ নাম আৰু আইডি হৈছে এৰেৰ সেই উপাদানৰ সূচী।
If we want to access the kth element in an array, we will use num[k-1] where num is the name of the array. kth element is present at index k-1.
যদি আমি এটা এৰেত কেথ উপাদানটো ব্যৱহাৰ কৰিব বিচাৰো, আমি সংখ্যা[কে-1] ব্যৱহাৰ কৰিম য’ত সংখ্যাটো হৈছে এৰেৰ নাম। কে.টি.এইচ. উপাদান সূচী কে-1-ত থাকে।
Each element can be treated as a single variable and we can use it the way we treat a variable – to take input from the keyboard, to display it or to use it for any operation.
প্ৰতিটো উপাদানক একক চলক হিচাপে গণ্য কৰিব পাৰি আৰু আমি ইয়াক আমি পৰিৱৰ্তনশীল এটাৰ চিকিৎসা কৰাৰ ধৰণে ব্যৱহাৰ কৰিব পাৰোঁ – কীবৰ্ডৰ পৰা ইনপুট ল’বলৈ, ইয়াক প্ৰদৰ্শন কৰিবলৈ বা যিকোনো অপাৰেচনৰ বাবে ব্যৱহাৰ কৰিবলৈ।
In the example considered in Figure 6.2, we have taken an integer type of array. Thus the following code segment will display the 3rd element of the array.
চিত্ৰ 6.2-ত বিবেচনা কৰা উদাহৰণত, আমি এক ইণ্টেগাৰ প্ৰকাৰৰ এৰে গ্ৰহণ কৰিছোঁ। এনেদৰে নিম্নলিখিত কোড খণ্ডটোৱে এৰেৰ তৃতীয় উপাদানটো প্ৰদৰ্শন কৰিব।

If we want to display all the elements of the array one by one, we can use a loop in the for (int index = 0; index < 7; index++) printf(“%d”, num[index]);
যদি আমি এৰেৰ সকলো বোৰ উপাদান এটা এটাকৈ প্ৰদৰ্শন কৰিব বিচাৰো, আমি (ইণ্ট ইণ্ডেক্স = 0; সূচক < 7; সূচী++) প্ৰিণ্টফ (“%ডি”, সংখ্যা[সূচী]);

The value of the variable index will go from 0 to 6 as the loop iterates. As a result, the printf() statement displays num[0], num[1],… num[6]..
চলক সূচকৰ মূল্য 0 ৰ পৰা 6 লৈ যাব যিহেতু লুপ ইটাৰেট। ফলস্বৰূপে, প্ৰিণ্টফ() বিবৃতিত সংখ্যা[0], সংখ্যা[1] ,… প্ৰদৰ্শন কৰা হয় সংখ্যা[6]।।
A complete C program is shown in Example 6.1. This program declares two arrays: num and state. The first one is an integer array and the second one is a char array. Both the arrays are declared with some initial values in their elements.]
উদাহৰণ 6.1-ত এটা সম্পূৰ্ণ চি প্ৰ’গ্ৰাম দেখুওৱা হৈছে। এই প্ৰ’গ্ৰামটোৱে দুটা এৰে ঘোষণা কৰে: সংখ্যা আৰু অৱস্থা। প্ৰথমটো হৈছে এটা ইণ্টেগাৰ এৰে আৰু দ্বিতীয়টো হৈছে এটা চাৰ এৰে। দুয়োটা এৰে তেওঁলোকৰ উপাদানবোৰত কিছুমান প্ৰাৰম্ভিক মূল্যৰ সৈতে ঘোষণা কৰা হয়।
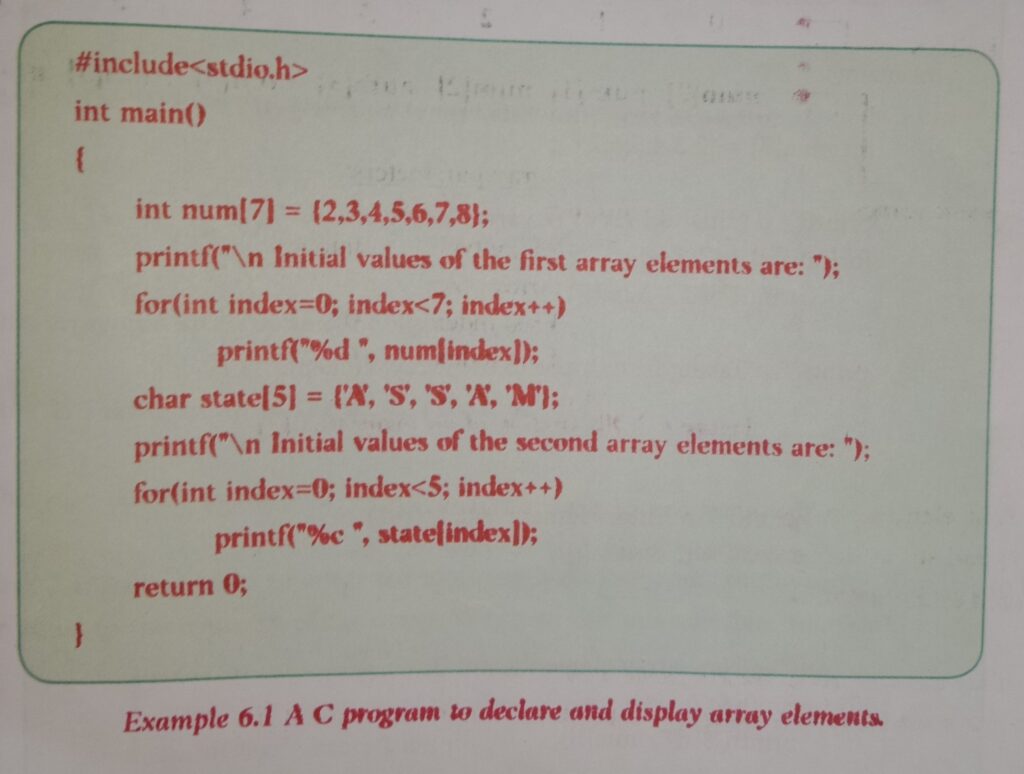
The first for loop was used to display the elements of the first array (line number 6 and 7). We used another for loop to display the elements of the second array (line number 9 and 10). As the elements of the first array are of type integer, we used %d format specifier to display. But the elements of the second array are of type character. So we used %c format specifier (line number 10).
The first for loop was used to display the elements of the first array (line number 6 and 7). We used another for loop to
প্ৰথম এৰেৰ উপাদানবোৰ প্ৰদৰ্শন কৰিবলৈ লুপৰ বাবে প্ৰথমটো ব্যৱহাৰ কৰা হৈছিল (শাৰী নম্বৰ 6 আৰু 7)। আমি দ্বিতীয় এৰেৰ উপাদানবোৰ প্ৰদৰ্শন কৰিবলৈ লুপৰ বাবে আন এটা ব্যৱহাৰ কৰিছিলো (শাৰী নম্বৰ 9 আৰু 10)। যিহেতু প্ৰথম এৰেৰ উপাদানবোৰ প্ৰকাৰৰ ইণ্টেগাৰৰ, আমি প্ৰদৰ্শন কৰিবলৈ %ডি ফৰ্মেট স্পেচিফায়াৰ ব্যৱহাৰ কৰিছিলো। কিন্তু দ্বিতীয় এৰেৰ উপাদানবোৰ প্ৰকাৰৰ চৰিত্ৰৰ। গতিকে আমি %চি ফৰ্মেট স্পেচিফায়াৰ (শাৰী নম্বৰ 10) ব্যৱহাৰ কৰিছিলো।
In the above code segments, we have learned another syntax of the C language. That is, we can declare a variable in the initialization portion of the for loop. Then we can use that variable within that loop. We did this in case of the variable index in the code segments.
ওপৰোক্ত কোড শাখাবোৰত, আমি চি ভাষাৰ আন এটা বাক্যবিন্যাস শিকিছোঁ। অৰ্থাৎ, আমি লুপৰ আৰম্ভণি অংশত এটা চলক ঘোষণা কৰিব পাৰোঁ। তেতিয়া আমি সেই লুপৰ ভিতৰত সেই চলকটো ব্যৱহাৰ কৰিব পাৰোঁ। কোড শাখাবোৰত পৰিৱৰ্তনশীল সূচকৰ ক্ষেত্ৰত আমি এইটো কৰিছিলো।
If we want to take input to the array from the keyboard, we can apply a similar strategy. For taking input to one particular element in the array (say, the 3rd element), we can use the following code segment.
যদি আমি কীবোৰ্ডৰ পৰা এৰেলৈ ইনপুট ল’ব বিচাৰো, আমি একে ধৰণৰ ৰণনীতি প্ৰয়োগ কৰিব পাৰোঁ। এৰেৰ এটা নিৰ্দিষ্ট উপাদানলৈ ইনপুট লোৱাৰ বাবে (কওঁক, তৃতীয় উপাদান), আমি নিম্নলিখিত কোড শাখাটো ব্যৱহাৰ কৰিব পাৰোঁ।
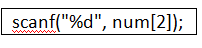
In case we wish to take input in the whole array, we scan the elements one by one using a loop. The following code segment can do this job.
যদি আমি গোটেই এৰেটোত ইনপুট ল’ব বিচাৰো, আমি লুপ এটা ব্যৱহাৰ কৰি এটা এটাকৈ উপাদানবোৰ স্কেন কৰোঁ। নিম্নলিখিত কোড খণ্ডটোৱে এই কামটো কৰিব পাৰে।

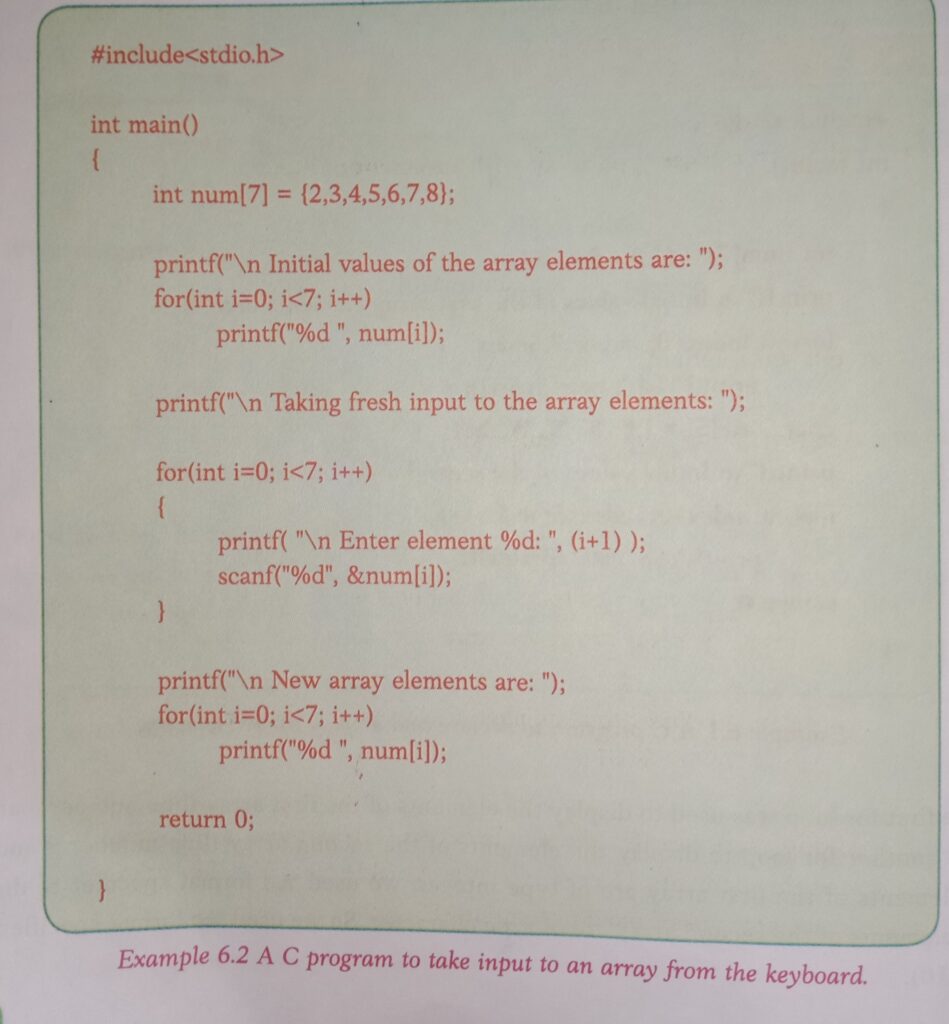
Example 6.2 shows a complete C program that displays the initial values of the array elements (line number 6 and 7), then takes input to all the array elements (line numbers 8 to 12) and then displays the new values (line number 14 and 15).
উদাহৰণ 6.2-এ এটা সম্পূৰ্ণ চি প্ৰ’গ্ৰাম দেখুৱায় যি এৰে উপাদানবোৰৰ প্ৰাৰম্ভিক মানবোৰ প্ৰদৰ্শন কৰে (শাৰী নম্বৰ 6 আৰু 7), তাৰ পিছত সকলো এৰে উপাদানলৈ ইনপুট লয় (শাৰী সংখ্যা 8 ৰ পৰা 12) আৰু তাৰ পিছত নতুন মানবোৰ প্ৰদৰ্শন কৰে (শাৰী নম্বৰ 14 আৰু 15)।