ফাংচনৰ 7.2 টা উপাদান
There are three parts of a function in C.
চি-ত এটা ফাংচনৰ তিনিটা অংশ আছে।
1. Function declaration: A function must be declared first before its use. This is to tell the compiler about the function name, function parameters, and return type. We gi
ve an example of a function declaration below.
ফাংচন ঘোষণা: এটা ফাংচন ব্যৱহাৰৰ আগতে প্ৰথমে ঘোষণা কৰিব লাগিব। এইটো ফাংচন নাম, ফাংচন প্ৰাচল, আৰু ঘূৰি অহাৰ প্ৰকাৰৰ বিষয়ে কম্পাইলাৰক জনোৱা। আমি তলত এটা ফাংচন ঘোষণাৰ উদাহৰণ দিওঁ।

2. Function call: This statement is responsible for the execution of a function. A function starts its execution when we make a call to it. A function can be called from anywhere in the program. The parameter list in function calling and function declaration must match. We must pass the same number of parameters as it is declared in the function declaration.
ফাংচন কল: এই বিবৃতিটো এটা ফাংচন সম্পাদন কৰাৰ বাবে দায়বদ্ধ। যেতিয়া আমি ইয়াক কল কৰোঁ তেতিয়া এটা ফাংচনে ইয়াৰ কাৰ্যকৰীকৰণ আৰম্ভ কৰে। প্ৰ’গ্ৰামৰ যিকোনো স্থানৰ পৰা ফাংচন এটা কল কৰিব পাৰি। ফাংচন কলিং আৰু ফাংচন ঘোষণাৰ প্ৰাচল তালিকা মিলাব লাগিব। আমি ফাংচন ঘোষণাত ঘোষণা কৰাৰ দৰে একে সংখ্যক মাপকাঠি পাছ কৰিব লাগিব।
The following code statement makes a call to the function “calculate_interest” and passes three parameters. The result of the function is stored in a variable “interest”.
নিম্নলিখিত কোড বিবৃতিটোৱে ফাংচনটোলৈ “calculate_interest” কল কৰে আৰু তিনিটা প্ৰাচল পাছ কৰে। ফাংচনৰ ফলাফলটো পৰিৱৰ্তনশীল “আগ্ৰহ”ত সঞ্চিত কৰা হয়।

3. Function definition: This portion of a function contains the actual statements that are executed when the function is called. The last statement in a function definition is the return statement that we wish to return to the caller of the function.
ফাংচন সংজ্ঞা: ফাংচন এটাৰ এই অংশত ফাংচনটো কোৱা হ’লে কাৰ্যকৰী কৰা প্ৰকৃত বিবৃতিবোৰ থাকে। ফাংচন সংজ্ঞাৰ অন্তিম বিবৃতিটো হ’ল ৰিটাৰ্ণ বিবৃতি যাক আমি ফাংচনৰ কলাৰৰ ওচৰলৈ ঘূৰি আহিব বিচাৰো।
The following code segment is the definition of a function calculate_interest(). The function calculates simple interest using the values of the parameters and returns it.
নিম্নলিখিত কোড খণ্ডটো হৈছে calculate_interest ফাংচন এটাৰ সংজ্ঞা()। ফাংচনটোৱে প্ৰাচলবোৰৰ মূল্যব্যৱহাৰ কৰি সৰল আগ্ৰহ গণনা কৰে আৰু ইয়াক ঘূৰাই দিয়ে।
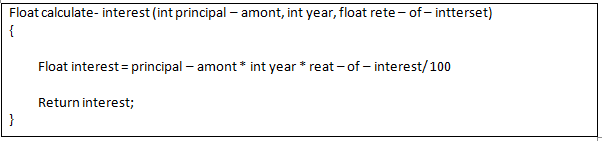
Figure 7.1 shows a simple C program that uses a function sum() to find the summation of two integers. The function accepts two integer parameters and returns another integer. In the program, main() is another function and it is calling a function sum() in line number 6. Thus main() can be called as a caller function and sum() can be called as a callee function.
চিত্ৰ 7.1-ত এটা সৰল চি প্ৰ’গ্ৰাম দেখুওৱা হৈছে যিদুটা পূৰ্ণসংখ্যাৰ সাৰাংশ বিচাৰিবলৈ ফাংচন যোগফল () ব্যৱহাৰ কৰে। ফাংচনটোৱে দুটা ইণ্টেগাৰ প্ৰাচল গ্ৰহণ কৰে আৰু আন এটা ইণ্টেগাৰ ঘূৰাই দিয়ে। প্ৰ’গ্ৰামটোত, মুখ্য() হৈছে আন এটা ফাংচন আৰু ই শাৰী নম্বৰ 6-ত ফাংচন যোগফল () বুলি কয়। এনেদৰে মুখ্য () কলাৰ ফাংচন বুলি ক’ব পাৰি আৰু যোগফল () কলি ফাংচন বুলি ক’ব পাৰি।.0
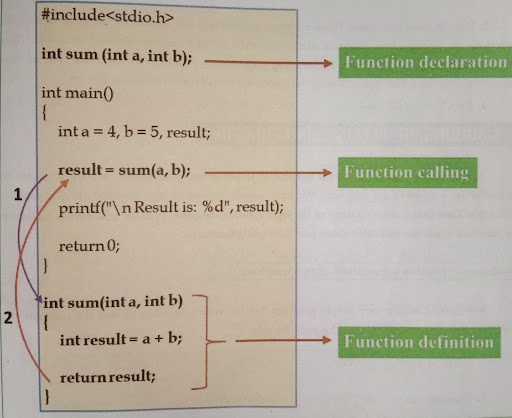
Function declaration and definition can also be written together. We will see examples of this later in this chapter.
ফাংচন ঘোষণা আৰু সংজ্ঞাএকেলগে লিখিব পাৰি। আমি এই অধ্যায়ৰ পিছত ইয়াৰ উদাহৰণ দেখিম।
There exists only one declaration and definition of a function. But a function may be called any number of times. Everytime we call a function, the control goes from the caller to the definition of the callee. After executing all the statements in the callee function definition, the control goes back to the caller. These are shown using arcs 1 and 2 in Figure 7.1.
এটা ফাংচনৰ কেৱল এটা ঘোষণা আৰু সংজ্ঞা আছে। কিন্তু ফাংচন এটাক যিকোনো বাৰ কোৱা হ’ব পাৰে। যেতিয়াই আমি ফাংচন এটা কওঁ, নিয়ন্ত্ৰণটো কলাৰৰ পৰা কেলিৰ সংজ্ঞালৈ যায়। কেলি ফাংচন সংজ্ঞাৰ সকলো বিবৃতি কাৰ্যকৰী কৰাৰ পিছত, নিয়ন্ত্ৰণটো ফোন কৰোতাৰ ওচৰলৈ ঘূৰি যায়। এইবোৰ চিত্ৰ 7.1-ত আৰ্ক 1 আৰু 2 ব্যৱহাৰ কৰি দেখুওৱা হৈছে।